Sidr
The best jQuery plugin for creating side menus and the easiest way for doing your menu responsive
You will be able to create multiple sidrs on both sides of your web to make responsives menus (or not, it works perfectly on desktop too).
It uses CSS3 transitions (and fallbacks to $.animate
with older browsers) and it supports multiple source types.
Get started
Like any other plugin, you must include it after the jQuery script. For a better performance load them at the bottom of your page or in an asynchronous way.
You have to include a Sidr Theme stylesheet too, choose between the dark or the light one, or if you prefer create one by your own.
<!DOCTYPE html>
<html>
<head>
<!-- Your other stuff (you can have problems if you don't add minimum scale in the viewport) -->
<meta name="viewport" content="width=device-width,minimum-scale=1">
<!-- Include a Sidr bundled CSS theme -->
<link rel="stylesheet" href="//cdn.jsdelivr.net/jquery.sidr/2.2.1/stylesheets/jquery.sidr.dark.min.css">
</head>
<body>
<!-- More stuff -->
<!-- Include jQuery -->
<script src="//cdn.jsdelivr.net/jquery/2.2.0/jquery.min.js"></script>
<!-- Include the Sidr JS -->
<script src="//cdn.jsdelivr.net/jquery.sidr/2.2.1/jquery.sidr.min.js"></script>
</body>
</html>
Using bower or NPM
Instead of downloading the plugin, you can install it with bower or with npm:
bower install sidr --save
npm install sidr --save
From a CDN
If you want to load the scripts from a CDN to save bandwith or improve the performance you can use jsDelivr.
Demos & Usage
Here are described differents ways of usage for this plugin, you can read and adapt them to your website’s requeriments. Below are described all options with details.
The Simplest Usage
Simple menuCreate a div called sidr and place your menu there. Then activate the plugin binding it to a link. By default, the menu wont’t be visible and it will be displayed or hidden by clicking on the link.
<a id="simple-menu" href="#sidr">Toggle menu</a>
<div id="sidr">
<!-- Your content -->
<ul>
<li><a href="#">List 1</a></li>
<li class="active"><a href="#">List 2</a></li>
<li><a href="#">List 3</a></li>
</ul>
</div>
<script>
$(document).ready(function() {
$('#simple-menu').sidr();
});
</script>
Create Multiple Menus
Left Menu Right MenuYou can create as many menus as you want in the same page, and you can place them at the right or left side. When creating more than one menu, you need to name them. As it is shown in the example, if you don’t create the menu div container, the plugin will create it for you.
<a id="left-menu" href="#left-menu">Left Menu</a>
<a id="right-menu" href="#right-menu">Right Menu</a>
<script>
$(document).ready(function() {
$('#left-menu').sidr({
name: 'sidr-left',
side: 'left' // By default
});
$('#right-menu').sidr({
name: 'sidr-right',
side: 'right'
});
});
</script>
The Menu Content
Existing content Load remotelly Callback loadedThere are four ways to load content in the menus, choose yours with the source option.
- We have shown the first way at the ‘Simplest Usage’ demo, no more than placing the content into the div menu.
- The most common way is to load existing html into the menu, you can add as many selectors as you want and they will be loaded in order.
- There is the possibility to load remote content easily via AJAX.
- If you need a more complex way to load content into the menu you can just create a callback function.
<a id="existing-content-menu" href="#existing-content-menu">Existing content</a>
<a id="remote-content-menu" href="#remote-content-menu">Load remotelly</a>
<a id="callback-menu" href="#callback-menu">Callback loaded</a>
<header id="demoheader">
<h1>Demos & Usage</h1>
</header>
<div id="demo-content">
<p>Here are described differents ways of usage for this plugin, you can
read and adapt them to your website's requeriments. Below are described
all options with details.</p>
</div>
<script>
$(document).ready(function() {
$('#existing-content-menu').sidr({
name: 'sidr-existing-content',
source: '#demoheader, #demo-content'
});
$('#remote-content-menu').sidr({
name: 'sidr-remote-content',
source: 'https://www.example.com/remote-menu.html'
});
$('#callback-menu').sidr({
name: 'sidr-callback',
source: function(name) {
return '<h1>' + name + ' menu</h1><p>Yes! You can use a callback too ;)</p>';
}
});
});
</script>
Responsive Menus
Responsive MenuThe major reason for creating this plugin was just being able to easily add existing content (like a menu, a search box, social icons,…) to a menu in small screens. Simply load existing html into a sidr, and then, hide this html and show the menu button with media queries.
<style>
#mobile-header {
display: none;
}
@media only screen and (max-width: 767px){
#mobile-header {
display: block;
}
}
</style>
<div id="mobile-header">
<a id="responsive-menu-button" href="#sidr-main">Menu</a>
</div>
<div id="navigation">
<nav class="nav">
<ul>
<li><a href="#download">Download</a></li>
<li><a href="#getstarted">Get started</a></li>
<li><a href="#usage">Demos & Usage</a></li>
<li><a href="#documentation">Documentation</a></li>
<li><a href="#themes">Themes</a></li>
<li><a href="#support">Support</a></li>
</ul>
</nav>
</div>
<script>
$('#responsive-menu-button').sidr({
name: 'sidr-main',
source: '#navigation'
});
</script>
Open/Close Programatically
There are some methods you can use to open or close menus as you want, or to bind them to any event. For example, in this page the right/left swipe touch event opens or closes the responsive menu (Note: this plugin doesn’t implement touch events, in this case I’m using an external library).
<!-- For this example I include an external library to handle touch events -->
<script src="//cdn.jsdelivr.net/jquery.touchswipe/1.6.15/jquery.touchSwipe.min.js"></script>
<script>
$('body').swipe( {
//Single swipe handler for left swipes
swipeLeft: function () {
$.sidr('close', 'sidr-main');
},
swipeRight: function () {
$.sidr('open', 'sidr-main');
},
//Default is 75px, set to 0 for demo so any distance triggers swipe
threshold: 45
});
</script>
Cookbook
You can see other recipes in the code repository:
https://github.com/artberri/sidr/tree/master/examples
Documentation
.sidr()
Description: It creates a sidr menu and binds the toggle function to the selector.
jQuery(selector).sidr( [options] )
options (Object)
A map of options to pass to the method.
name (String) Default: 'sidr'
Name for the sidr.
side (String) Default: 'left'
Left or right, the location for the sidebar.
source (String|Function) Default: null
A jQuery selector, an url or a callback function.
renaming (Boolean) Default: true
When filling the sidr with existing content, choose to rename or not the classes and ids.
body (String) Default: 'body' [ Version 1.1.0 an above ]
For displacing the page the 'body' element is animated by default, you can select another element to animate with this option.
displace (Boolean) Default: true [ Version 1.2.0 an above ]
Displace the body content or not.
timing (String) Default: 'ease' [ Version 2.1.0 an above ]
Timing function for CSS transitions.
method (String) Default: 'toggle' [ Version 2.1.0 an above ]
The action to execute when clicking the button. 'toggle', 'open' and 'close' are allowed.
bind (String) Default: 'touchstart click' [ Version 2.2.0 an above ]
The event(s) to trigger the menu. Only 1 event will be triggered each 100ms, so only the first one will be triggered if there are 2 at the same time.
onOpen (function) Default: function() {} [ Version 1.2.0 an above ]
Callback that will be executed when the menu starts opening.
onOpenEnd (function) Default: function() {} [ Version 2.1.0 an above ]
Callback that will be executed when the menu ends opening.
onClose (function) Default: function() {} [ Version 1.2.0 an above ]
Callback that will be executed when the menu starts closing.
onCloseEnd (function) Default: function() {} [ Version 2.1.0 an above ]
Callback that will be executed when the menu ends closing.
jQuery.sidr()
Description: A generic sidr controller. Can be used to access the sidr methods: open, close, toggle or status
jQuery.sidr( [method] [, name] [, complete] )
method (String) Default: 'toggle'
Choose between 'toggle', 'open', 'close' or 'status'. [ Status is only available in version 2.1.0 an above ]
name (String) Default: 'sidr'
Name of the target sidr.
complete (Function) Default: none
A function to call once the animation is complete.
return null || Object [ Version 2.2.0 an above ]
It returns null when methods 'toggle', 'open' or 'close' are called, or an object when status is called:
{ moving: (boolean), // A boolean indicating if the menu is currently being moved opened: (string || false) // Name of the opened menu or false if all are closed }
Themes
There are two themes (two stylesheets) included with the plugin, a dark one and a light one. You can use them, create a new one or override them with your own styles.
Showcase
The following responsive sites are using Sidr in an original way to implement their menu:
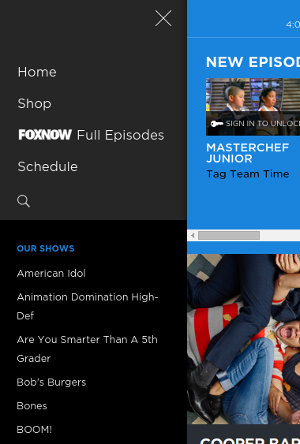
FOX Broadcasting Company

United States Courts

San Diego State University
If you’ve created a website or an application using this plugin and you want to show it in this section, send me an email with the url to [email protected].
Development
- Source hosted at GitHub
- If you have problems implenting this, ask about it in StackOverflow
- Report issues and feature requests in GitHub Issues
- Contributing: CONTRIBUTING.md
Pull requests are very welcome! Make sure your patches are well tested. Please create a topic branch for every separate change you make.